Track
Python lists stand as a fundamental data structure essential for storing and manipulating collections of items. Their flexibility and ease of use make them indispensable in a wide range of programming tasks, from data analysis to web development. Given their pervasive use, a common requirement that emerges is determining the size of a list. While the len() function is widely known, Python's rich syntax and library support offer multiple other ways to achieve this, each with its own nuances and applications. This article delves into eight such methods, providing insights into their workings and efficiency.
Why Are Python Lists Important?
Lists in Python are dynamic arrays that can hold a mix of data types, offering a versatile tool for data storage and manipulation. They facilitate operations such as iteration, slicing, and comprehensive manipulations with built-in methods like append(), remove(), and sort(), among others. You can read more about list manipulations in this tutorial.
How to Define a List in Python
For the purposes of this tutorial, we will use a simple list to demonstrate each method. Consider the list my_list
, defined as follows:
# Define a sample list to be used throughout the tutorial
my_list = ["I", "Love", "Learning", "Python"]
This list, albeit simple, serves as a perfect candidate to illustrate various techniques for determining its length.
8 Ways to Find the Python List Size
1. Find the Size of a List Using the len()
Function
The len()
function is the most straightforward approach to ascertain the size of a list. It is not only concise but also highly efficient, making it the go-to method in most cases.
# Use len() to find the size of the list
length = len(my_list)
print(length) # Output: 4
2. Find the Size of a List Using a For Loop
Referred to as the naive method, this approach involves initializing a counter and incrementing it for each element encountered in a for loop through the list. You can learn more about for loops and other types of loops in this tutorial.
# Naive method using a for loop to count the list's size
counter = 0
for item in my_list:
counter += 1
print(counter) # Output: 4
3. Find the Size of a List Using a List Comprehension
List comprehensions in Python offer a compact syntax for performing operations on a list. Here, we use it alongside the sum()
function to count the elements.
# Using list comprehension to count the list's size
length = sum([1 for item in my_list])
print(length) # Output: 4
4. Find the Size of a List Using reduce()
The reduce()
function is a bit more advanced but very powerful. It applies a function of two arguments cumulatively to the items of a list, from left to right, so as to reduce the list to a single value. For our purpose, we'll use it to count the number of items in a list.
To make this easier to understand, we'll break it down into two parts:
- Define a Simple Function: First, we'll define a simple function that takes two arguments: the current count (which we'll call
count_so_far
) and an individual item from the list (which we'll name_
to indicate that we won't actually use this item). - Use
reduce()
: Then, we'll usereduce()
to apply this function to every item in the list, effectively counting the items as it goes.
# Import the reduce function from the functools module
from functools import reduce
# Define a simple function to use with reduce
def update_count(count_so_far, _):
"""Increases the count by 1. The second parameter is not used."""
return count_so_far + 1
# Use reduce to count the items in the list
# We start counting from 0, which is why we have '0' at the end
list_length = reduce(update_count, my_list, 0)
# Print out the result
print(list_length) # Output will be 4
5. Find the Size of a List Using iter()
and next()
Understanding how to count the elements in a list using iter()
and next()
involves getting familiar with iterators. An iterator is an object in Python that allows us to go through all the elements in a list one by one. The iter()
function turns a list into an iterator, and the next()
function moves to the next element in the iterator.
Here's a step-by-step breakdown:
- Create an Iterator: We start by turning our list into an iterator using the
iter()
function. This allows us to go through the list one item at a time. - Count Using a Loop: We set up a loop that will keep running until there are no more items in the list. Inside the loop, we use the
next()
function to move to the next item in the list. Each time we successfully move to the next item, we increase our count by 1. - Handle the End of the List: Eventually, we'll reach the end of the list. When that happens,
next()
will cause aStopIteration
error, indicating there are no more items. We catch this error with a try and except block and stop counting.
Here's the simplified code with comments to help understand each step:
# Step 1: Turn the list into an iterator
list_iterator = iter(my_list)
# Initialize a counter to keep track of the number of items
count = 0
# Step 2: Loop through the list using the iterator
while True:
try:
# Use next() to get the next item from the iterator
next(list_iterator)
# If next() was successful, increase the count
count += 1
except StopIteration:
# Step 3: If we reach the end of the list, break out of the loop
break
# Print out the total count of items in the list
print(count) # Output will be 4
6. Find the Size of a List Using enumerate()
The enumerate()
function in Python adds a counter to an iterable and returns it in a form of an enumerate object. This function is commonly used in loops to get both the index and the value of each item in the list. While enumerate()
is not typically used to find the size of a list, we can use it to illustrate how flexible Python can be.
# Step 1: Enumerate the list and convert it to a list of tuples (index, element)
enumerated_list = list(enumerate(my_list))
# Step 2: Extract the last tuple (which contains the last index and the last element)
last_tuple = enumerated_list[-1]
# Step 3: The size of the list is the last index plus 1 (because of zero-based indexing)
list_size = last_tuple[0] + 1
# Print out the size of the list
print(list_size) # Output will be 4
7. Find the Size of a List Using NumPy
NumPy is a powerful library in Python that is widely used in the field of scientific computing. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. For those working with numerical data, NumPy is an indispensable tool, offering efficient storage and operations on large datasets. To find the size of a list in Python using NumPy, we can use the .size
attribute as shown below.
# Import the NumPy library
import numpy as np
# Step 1: Convert the list into a NumPy array
my_array = np.array(my_list)
# Step 2: Use the 'size' attribute of the NumPy array to find its size
array_size = my_array.size
# Print out the size of the array (which is the same as the length of the list)
print(array_size) # Output will be 4
8. Find the Size of a List Using map()
and sum()
Similar to list comprehension, this method uses map()
to apply a function that returns 1 for each element, with sum()
, then aggregating these values to count the elements. This method requires familiarity with lambda functions in Python. To get up to speed, make sure to check out this Python lambda tutorial.
# Using map() and sum() to count the list's size
length = sum(map(lambda x: 1, my_list))
print(length) # Output: 4
Comparing the Performance of Different Python List Size Methods
It’s one thing to know all eight methods for finding the size of lists, but it’s another thing to use the best method. To compare the performance of the eight methods discussed, we'll use Python's timeit
module, which provides a simple way to time small bits of Python code. This will give us a clear idea of how each method stacks up in terms of execution speed. Note that execution times may differ depending on your machine or where you run your code.
Setting up the experiment
To make it easy on ourselves, we define our list and the functions for each method. It’s okay if you are not familiar with functions; all we are doing here is systematizing the different methods to make them easier to compare.
import timeit
from functools import reduce
import numpy as np
# Define the list
my_list = ["I", "Love", "Learning", "Python"] * 100 # Increased size for better statistical signifcance
# Method 1: Using len()
def method_len():
return len(my_list)
# Method 2: Looping through the list
def method_loop():
counter = 0
for _ in my_list:
counter += 1
return counter
# Method 3: Using a list comprehension
def method_list_comprehension():
return sum([1 for _ in my_list])
# Method 4: Using reduce()
def method_reduce():
return reduce(lambda acc, _: acc + 1, my_list, 0)
# Method 5: Using iter() and next()
def method_iter_next():
iterator = iter(my_list)
counter = 0
while True:
try:
next(iterator)
counter += 1
except StopIteration:
break
return counter
# Method 6: Using enumerate()
def method_enumerate():
return max(enumerate(my_list, 1))[0]
# Method 7: Using numpy
def method_numpy():
np_array = np.array(my_list)
return np_array.size
# Method 8: Using map() and sum()
def method_map_sum():
return sum(map(lambda _: 1, my_list))
Timing Each Method
Next, we'll use timeit.timeit()
to time each function according to the specified order:
# List to hold method names and their execution times
timing_results = []
methods = [method_len, method_loop, method_list_comprehension, method_reduce,
method_iter_next, method_enumerate, method_numpy, method_map_sum]
# Time each method
for method in methods:
# Execute the operation 100000 times for better statistical significance
time_taken = timeit.timeit(method, number=100000)
timing_results.append((method.__name__, time_taken))
# Sort results by time taken for better readability
timing_results.sort(key=lambda x: x[1])
# Print the timing results
for method_name, time_taken in timing_results:
print(f"{method_name}: {time_taken:.5f} seconds")
By running this in DataLab, we receive the following results:
Method |
Time taken |
The |
0.03410 seconds |
The list comprehension method |
1.50325 seconds |
The for loop method |
1.93764 seconds |
The |
2.26446 seconds |
The |
2.41315 seconds |
The |
3.08434 seconds |
The |
4.41239 seconds |
The numpy method |
10.61716 seconds |
Conclusion
In conclusion, this exploration into various methods for finding the size of a list in Python has not only showcased the versatility and power of Python as a programming language but also highlighted the importance of understanding the efficiency and applicability of different approaches.
For beginners, it's crucial to start with the most straightforward and efficient methods, such as using the built-in len() function, which is optimized for performance and readability. For more on working with lists, check out the following resources:
- Python Fundamentals skill track
- Introduction to Python course
- Python Cheat Sheet for Beginners
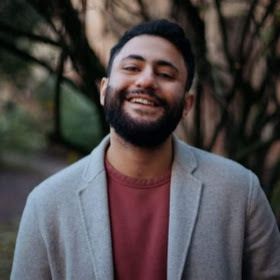
Adel is a Data Science educator, speaker, and Evangelist at DataCamp where he has released various courses and live training on data analysis, machine learning, and data engineering. He is passionate about spreading data skills and data literacy throughout organizations and the intersection of technology and society. He has an MSc in Data Science and Business Analytics. In his free time, you can find him hanging out with his cat Louis.
Start Your Python Journey Today!
Course
Introduction to Python for Finance
Track
Python Developer
Exploring Matplotlib Inline: A Quick Tutorial
How to Use the NumPy linspace() Function
Python Absolute Value: A Quick Tutorial
How to Check if a File Exists in Python
Writing Custom Context Managers in Python
Bex Tuychiev